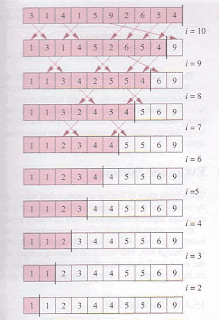
Scenario
I would like to sort an array collection of objects wherein some of them have property of string values that are usually empty. My requirements dictate that these empty values should be sorted on the end of the list.
The problem is, empty values goes to the beginning of the list by default when sorting.
Analysis
Using the SortField and Sort class we can sort objects in an ArrayCollection based on a specific property:Example
// Treat this an example ArrayCollection // var arrColl:ArrayCollection = new ArrayCollection([{number:3, name:''}, {number:2, name:'James'}, {number:1, name:'Aaron'}]); // Now passing this collection to a sorting function that sorts by name or number private function sortArrayCollection(arr:ArrayCollection, isDescending:Boolean = true, byNumber:Boolean = true):ArrayCollection { var dataSortFieldNumber:SortField = new SortField; dataSortFieldNumber.name ="number"; dataSortFieldNumber.numeric = true; dataSortFieldNumber.descending = isDescending; var dataSortFieldName:SortField = new SortField; dataSortFieldName.name ="name"; dataSortFieldName.numeric = false; dataSortFieldName.descending = isDescending; var dataSort:Sort = new Sort(); if(byNumber) dataSort.fields = [dataSortFieldNumber]; else dataSort.fields = [dataSortFieldName]; arr.sort = dataSort; arr.refresh(); return arr; }
With this the number and name can be sorted depending on which arrangement you choose. The problem here is when the name is arranged, it would appear as follows:
[Sample arrangement in ascending order of names] {number:3, name:''} {number:1, name:'Aaron'} {number:2, name:'James'}
As you can see, empty strings are always place at the beginning when arranged and vice-versa. By default, sorting arrange strings by this format (if ascending, and reversed if descending):
Empty String - alphabetically or depending[A-Z][a-z] - NULL
If you will not manipulate the string data, you can use the default arrangement to resolve the issue between the placement of emptyStrings and NULL values. Other than that you can also use the compareFunction to override the default sorting function.
No comments :
Post a Comment